Pyton 3 Quick Notes
Not backwards-compatible with Python 2. Dynamic typing. Variables are by convention lowercase and snake_case instead of camelCase. It makes sense for Phython to be snake_case (Ha Ha). No hyphens in the variable name.
See PEP-8 for style guide. Indentation signification (usually 4 spaces). Installation of autopep8 from PyPI to auto-format your code…
pip install autopep8
autopep8 –in-place filename.py
# two levels of aggressiveness changing more than just whitespace…
autopep8 –in-place -a -a filename.py
Python Comments
# This is a Python comment
There is no multi-line commens in Python.
Python Data Types (not all)
int
float
str
bool # True or False (capitalized)
NoneType # None
list
dict
Common Python Operators
% # modulo
// # integer division (truncates remainder)
Logical operators:
and
or
not
!=
==
is
Python Swapping
a = ‘alpha’
b = ‘beta’
a, b = b, a
Common Python functions
print()
len()
type()
int()
str()
float()
input()
dir() # prints out the attributes of an object
all([0, 1, 2, 3]) # False
any([0, 1, 2, 3]) # True
Common Python String Functions
msg = “Hello ” + “World”
answer = f”He said: {msg}”
“, “.join(my_list)
something.upper()
something.lower()
my_str = r”\bHello\b” # raw string for regular expressions
Python Assertions
Taking the previous example, we can use Python assertion to make sure we get what we get.

If we don’t get what we expect, the program will stop with an AssertionError.
Do not rely on assertions for your program to behave as you intend. Because assertions can be turned off with the -O option in the python command line.
Python List
fruits = [“apple”, “pear”, “orange”] # order and can have dups
for fruit in fruits:
print(fruit)
fruits[0] # ‘apple’
fruits[1:] # [‘pear’, ‘orange’]
# fruits[start:end:step]
fruits[-1] # ‘orange’
fruits.index(“pear”) # 1
fruits.index(“pear”, 0, len(fruits)) # equivalent to above
# start/end index not including ending index
fruits.index(“cat”) # valueError
fruits.count(“pear”) # 1
fruits.count(“hat”) # 0
fruits.insert(2, “banana”)
fruits.insert(-1, “kiwi”)
# [‘apple’, ‘pear’, ‘banana’, ‘kiwi’, ‘orange’]
fruits.pop() # returns “orange”
# [‘apple’, ‘pear’, ‘banana’, ‘kiwi’]
fruits.pop(1) # returns “pear”
# [‘apple’, ‘banana’, ‘kiwi’]
fruits.remove(“banana”) # returns None
# [‘apple’, ‘kiwi’]
# valueError if not found
fruits.append(“grapes”) # adds only one
fruits.extend([“avocado”, “papaya”])
fruits.sort()
fruits.reverse()
del fruits[1:2]
fruits.clear()
nums = [1, 7, 8, 13, 2, 5, 8, 9, 1]
nums[1:4:2] # [7, 13]
Python For Loops
# prints 1 to 10
for i in range(1,11):
print(i)
# prints 0 to 3
for i in range(4):
print(i)
# prints 2, 4, 6, 8
for i in range(2, 10, 2):
print(i)
Python While Loops
# print 5,4,3,2,1
i = 5
while i > 0:
print(i)
i -= 1
Python List Comprehension
nums = [1, 2, 3]
double = [ num * 2 for num in nums] # [2, 4, 6]
answer = [ num * 2 for num in nums if num % 2 == 0] # [4]
answer = [‘even’ if num % 2 == 0 else ‘odd’ for num in nums]
# [‘odd’, ‘even’, ‘odd’]
Python Dictionary
dict(a=1, b=2, c=3)
dict([ [‘a’, 1], [‘b’, 2], [‘c’, 3] ])
user = { 'first name': 'Hello',
'last name': 'World',
'age': 9,
7: "lucky number" }
user['age'] # 9
user['junk'] # KeyError
user.get('age') # 9
user.get('junk') # None
user.keys()
user.values()
for key, value in user.items():
print(key, value)
"first name" in user # True
9 in user.values() # True
cloned_user = user.copy()
user.pop('age') # return 9 and removes key
# KeyError if no key
user.popitem() # removes something at random (‘last name’, ‘World’)
user.clear()
new_user = {}.fromkeys([‘first name’, ‘last name’, ‘age’], None]
new_user = dict.fromkeys([‘first name’, ‘last name’, ‘age’], None]
dict.fromkeys(range(0,10), 0)
first.update(second)
Python Dictionary Comprehension
nums = dict( first = 1, second = 2, third = 3)
double = { key : value * 2 for key, value in nums.items() }
answer = { key : ( ‘even’ if value % 2 == 0 else ‘odd ) for key, value in nums.items() }
Python Tuples
nums = (1, 2, 3, 4, 5) # immutable
nums = 1, 2, 3, 4, 5
nums = tuple([1,2,3,4,5])
3 in nums # True
new_nums = (*nums, 6)
for num in nums:
print(num)
empty = ()
singleton = (‘hello’,)
singleton = ‘hello’, # note the comma
Python Sets
my_set = { 7, 8, 10 } # no order and no duplicates
my_set = set({7, 8, 10})
my_set = set([7, 8, 10, 10]) # turn list to set removing dups
my_list = list(my_set) # turn set to list
7 in my_set # True
my_set.add(4)
my_set.remove(4)
my_set.remove(77) # throw KeyError if not exist
my_set.discard(77) # no error
for value in my_set:
print(value)
my_set.clear()
my_set.copy()
Union set: my_set | your_set
Intersect set: my_set & your_set
Set with even numbers:
{ x * 2 for x in range(10) }
Python Functions
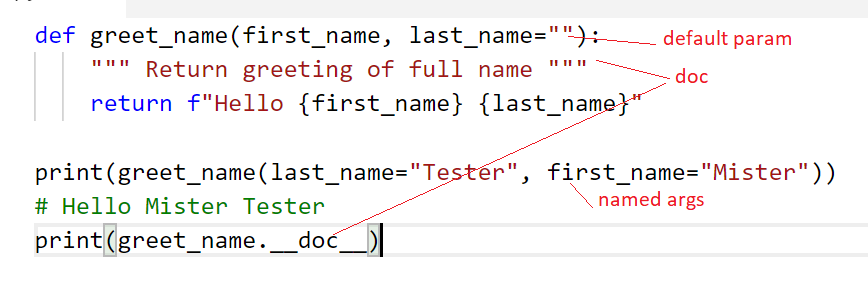
global variable
my_int = 3
def my_function():
global my_int
my_int += 4
print(my_int)
my_function()
# 7
nonlocal
def my_outer_function():
def my_function():
nonlocal my_int
my_int += 4
return (my_int)
my_int = 3
return my_function()
print(my_outer_function()) # 7
*args
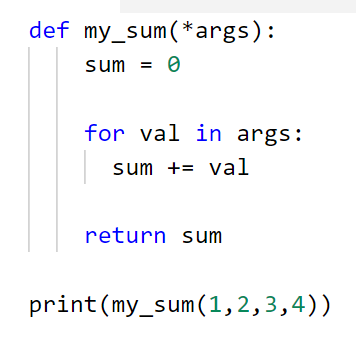
**kwargs
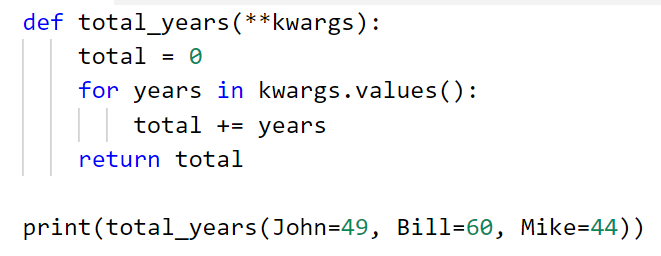
dictionary unpacking
But if your input is in the form of a dictionary instead of key-value pair, but the function is expecting key-value pair, do dictionary unpacking with ** like this …

tuple unpacking
Similarly if function parameter is expecting to be individual arguments, but you have a list or a tuple, unpack the tuple with single-star (*) when passing the argument.
Python Exception Handling
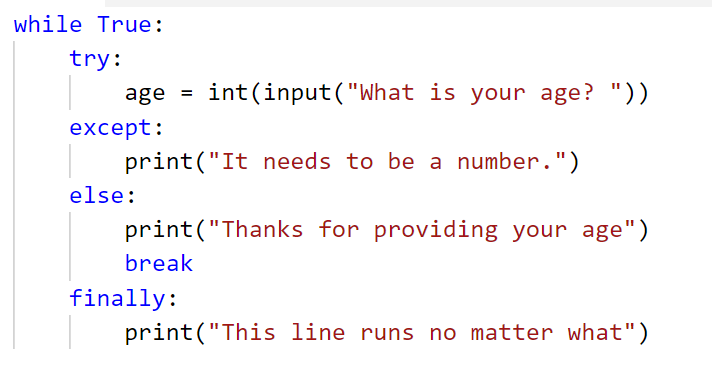
Because int() will raise an “ValueError” when input is not a number, we can specifically catch that type of error by …
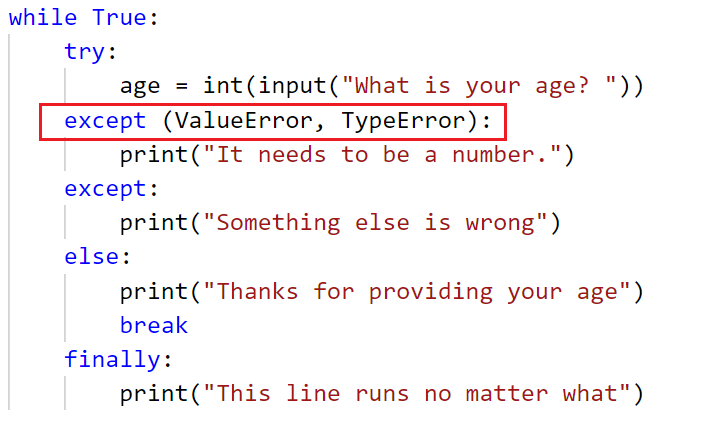
You can also “raise” built-in or custom exceptions derived from BaseException…
raise TypeError("your error message here")
Python Modules
import random
import random as rand
from random import *
from random import randint, choice
Python More Advance Topics
- Python debugging with pdb
- Lambda
- http requests for json
- Python classes
- Using regular expression in Python
- Iterable versus iterator
- Generators
- Reading Writing Files
- Reading CSV
- Serializing objects
- Parsing HTML elements
- Python Testing