Tutorial Python Star Operator
Python’s star operator is used in many context. The simplest one is the multiplication operator …
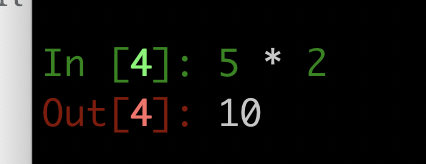
And the powers operator…
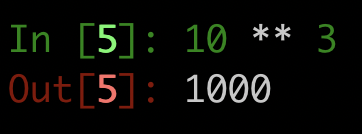
It is used for extending lists…


And similarly for strings …
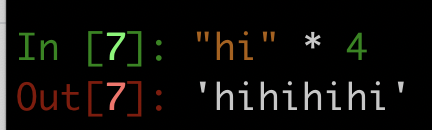
You can think of this a multiplication of strings and lists.
It is used for unpacking …
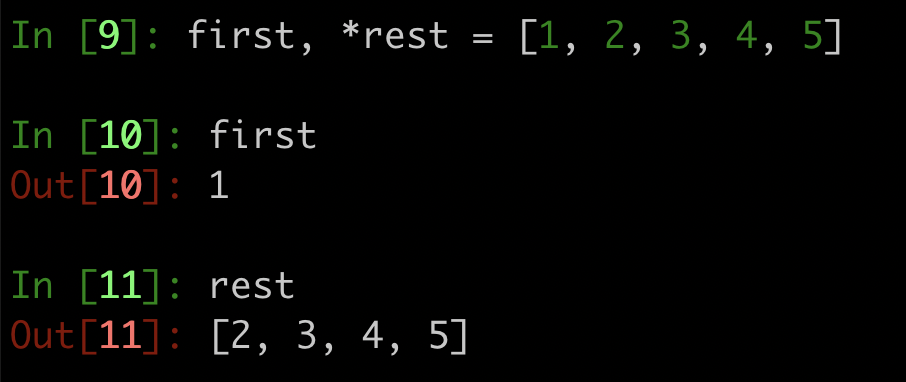
It is used for “spreading”. For example, suppose you have a function that takes three parameters…
def add(x, y, z):
return x + y + z
You would normally call it with three argument like this…
add(1, 2, 3)
But what if you don’t have three argument, but only one list with three items. You can have the three items in the list be spread out into three arguments like this…
add(*my_numbers)
And finally, the Python star operator is used in *args and **kwargs …
def example(*args, **kwargs):
print(args)
print(kwargs)
example(1, 2, 3, name="John", age=42)
# (1, 2, 3)
# {'name': 'John', 'age': 42}
The *args will collect all the positional arguments passed in and will be a tuple. The **kwargs will collect all the named arguments and will be a dictionary.