Server and Client side example of WebSockets using Node
In this example, we will implement WebSockets in Node server side first. And then we will create client-side HTML page to connect to that WebSocket via Javascript so that we can get a two-way communication.
WebSockets example in Node
Start with a bare bones Node server app (by following the steps in tutorial here). Your node index.js should look like this…
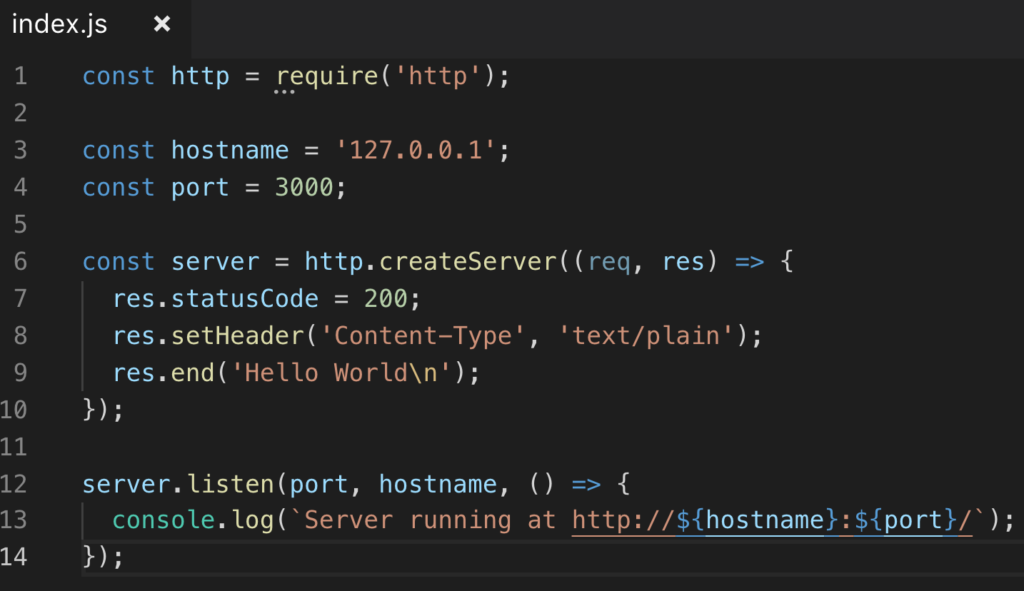
Now typing into your terminal …
node index.js
will start up your Node web server on port 3000 which returns “Hello World” when you point your browser to http://localhost:3000
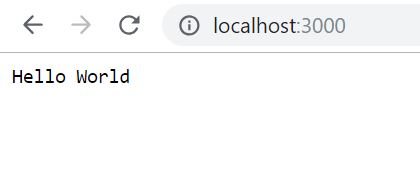
Next, we will add WebSockets on port 8080. To help us with this, we will use the Node package “ws” (https://www.npmjs.com/package/ws) which we install by …
npm install --save ws
After installation, we add the following code to our index.js file so that it looks like this …
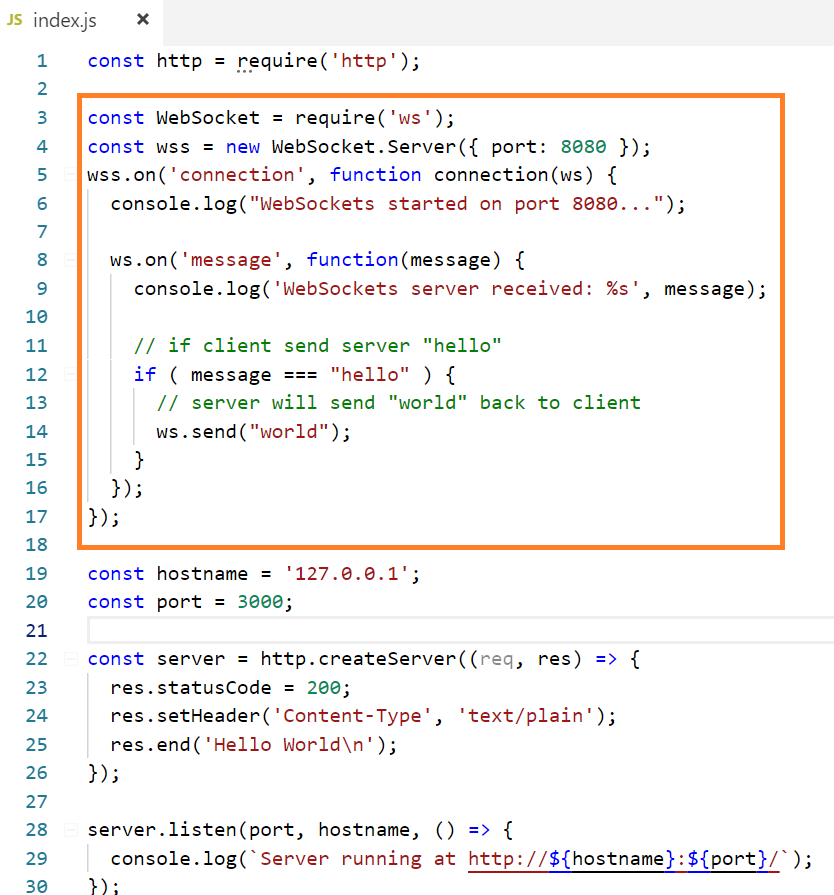
This WebSockets code was based on the “simple server” version of the example code found on the npm ws package page.
On line 3, we “require” the “ws” module that was just installed.
On line 4, we created a new WebSockets Server on port 8080.
Line 5 says that if server gets a connection from a client, then we set up a “message” event handler on line 8.
The message event handler will run every time a client sends the server a message. In line 12, we say that if client sends the message “hello”, the server will send back the word “world”.
Client-Side WebSockets example
Now lets create the client-side HTML page named “index.html” that will connect to your Node WebSockets connection.
From a basic HTML5 page, we add in the Javascript code as follows…
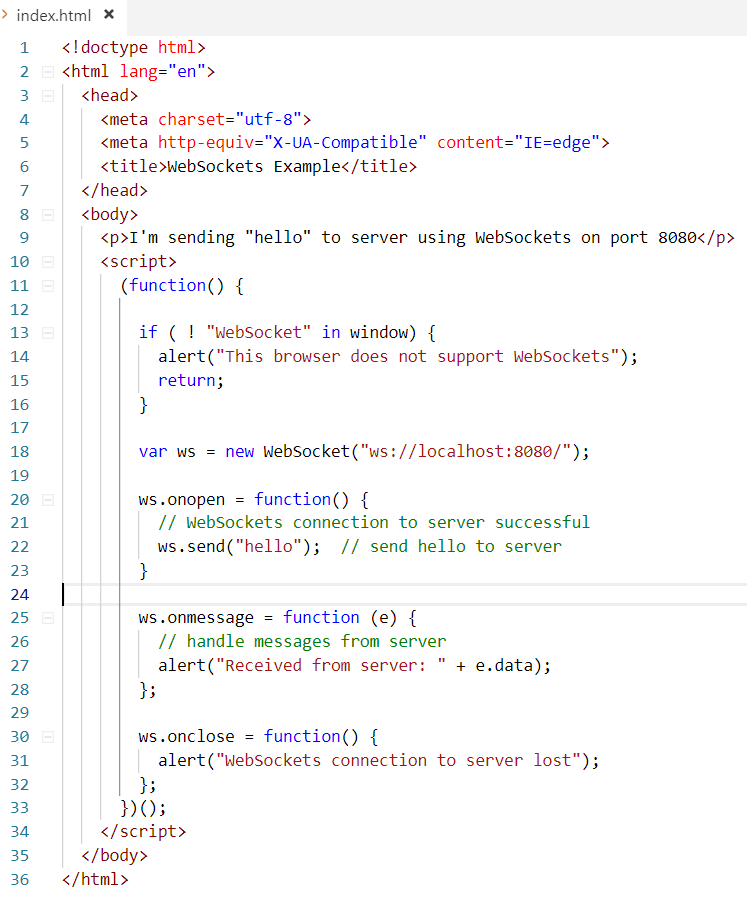
Line 11 and line 33 is just the enclosing IIFE (Immediately-invoked Function Expression).
Line 13 – 16 will terminate the function if browser does not support WebSockets. All modern web browser should be supporting WebSockets now.
Line 18 we connect to the server via port 8080.
On successful connection, we send to the server “hello” on line 22.
Whenever we receive something back from the server, we run “onmessage” event handler which simply “alert out” what the server sent.
Upon lost connection with WebSockets, we alert out line 31.
Start Node Server WebSockets
Restart your Node server with …
node index.js
Your Node server is now listening for WebSockets connection on port 8080. But since there was no client connecting to it yet, it did not console log out line 6.
The server terminal looks like this …
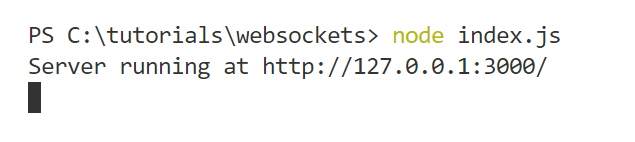
Keep it running for now.
Start Client
Open the index.html file in your browser …
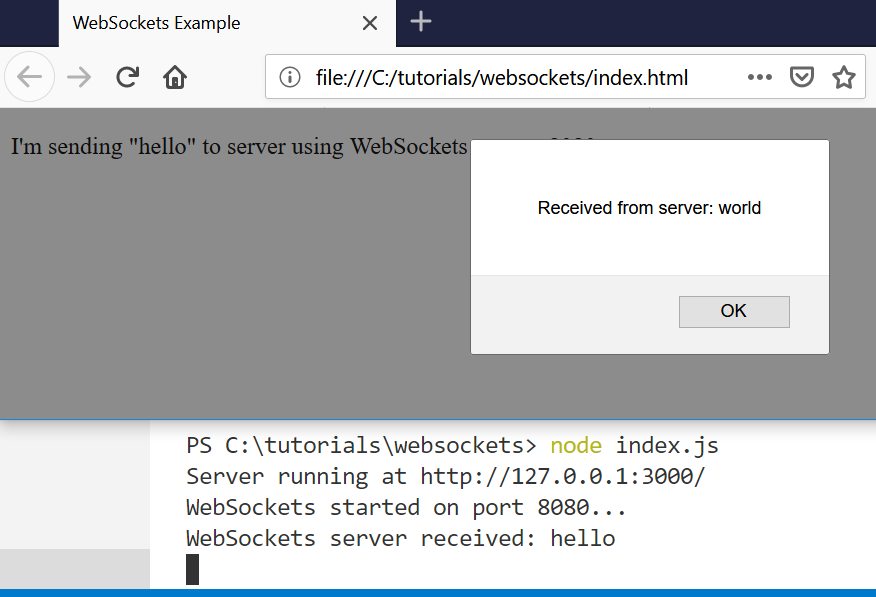
Immediately upon page load, Javascript connected to server. In the server terminal, you see “WebSockets start on port 8080…”
Next the client Javascript send message “hello” to the server.
In server terminal, you see “WebSockets server received: hello”.
Then server sends “world” to the client using WebSockets. Upon the detection of this incoming message, the client Javascript fires the “onmessage” event handler and alerts out the dialog you see in screenshot above. So client received the message.
Click “ok” to the client alert box. And you get back your index.html webpage.
Now on the server terminal, terminal the WebSockets with Ctrl-C. You see that the client detected lost connection and alert the dialog below…
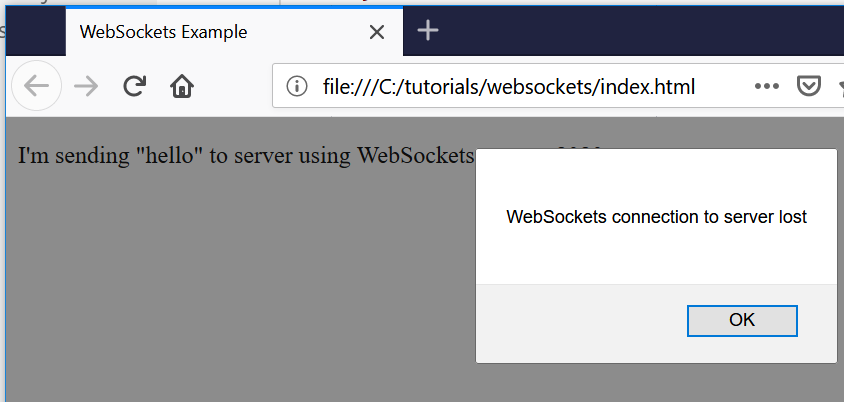
Code Sample
Linked here is the zipped of the code in this tutorial.