Use JQuery to Highlight Values in a Table
Suppose we have a table with some numbers. We want to use JQuery to highlight those numbers that are negative by coloring them in red and bolding them as shown …
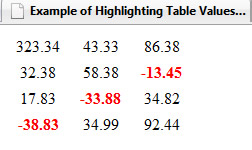
highlighted negative values
Here is our HTML and CSS code for the table …
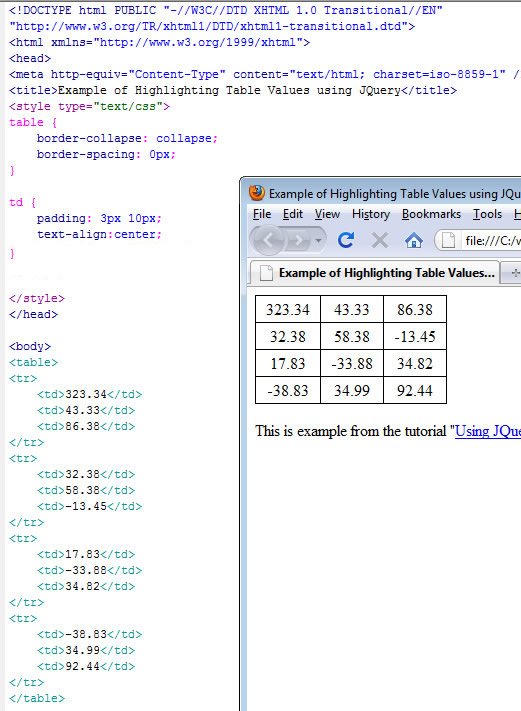
HTML and CSS for table
1. We will be putting our JQuery code in a file called “highlightvalues.js”. And we will be using the JQuery library from Google’s CDN (Content Delivery Network). So we reference both scripts in the head of our page…
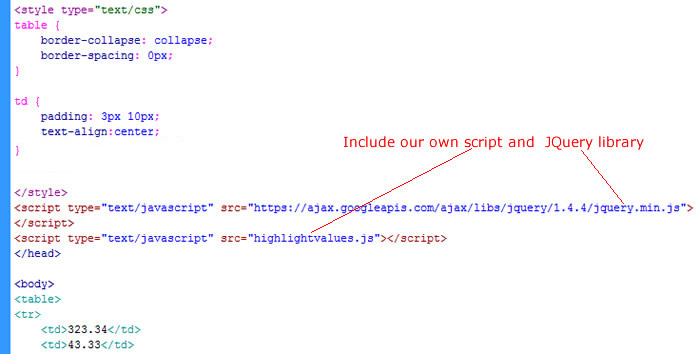
include JQuery script
See JQuery Tutorial on Table Styling for more details on including JQuery scripts.
2. In our highlightvalues.js file, put the following code …
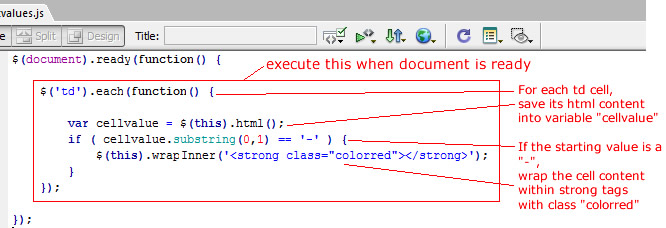
JQuery to code highlight negative values
The typical JQuery skeleton …
$(document).ready(function() {
...
});
ensures that our code inside it run only when the page document is loaded and ready.
Our code here …
$('td')
selects all td table cells.
And for each of these cells (that’s what the “.each” means), execute the following …
var cellvalue = $(this).html(); if ( cellvalue.substring(0,1) == '-' ) { $(this).wrapInner('<strong style="colorred"></strong>'); }
The $(this) refers to the currently selected cell (the td element). The html() function retrieves the HTML content of the cell. We save this in a variable called “cellvalue”.
We use the Javascript substring function to extract the first character (from character index 0 to index 1). If this character is a negative sign “-“, then execute …
$(this).wrapInner('<strong style="colorred"></strong>');
The wrapInner() function will put the “strong” tags around the cell content. When we run our code and examine it in FireBug, we see the strong tags have been dynamically inserted around our cell content as shown below.
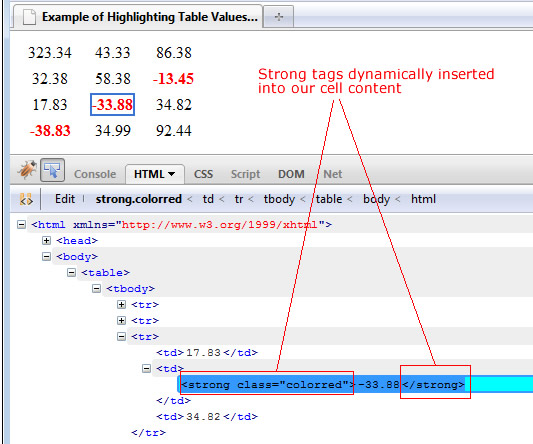
inserted tags into cell content
3. You may not see the numbers as being colored red yet. That is because although the strong tag has the class=”colorred” attribute, we still need to add this class rule in the CSS stylesheet …
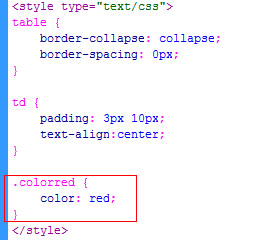
add CSS style rule
After adding that you should see the negative value become red as shown…
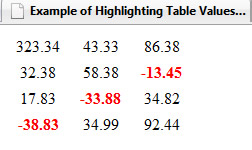
highlighted negative values
See live code example.
The reason that we use wrapInner() instead of wrap() is because we want to wrap the “strong” tags around our cell content instead of wrapping the strong tag around our td element (which would be what wrap() would have done since the “$(this)” refers to the td).