Difference between Javascript and Python class
In previous tutorials, we wrote a class in Javascript and in Python.
Refer back to those tutorial codes and you will see that both uses the “class” keyword to define a class.
In Javascript, the constructor is a constructor function and is not preceded by the “function” keyword. It takes an initialization parameter which it saves to a property attached to the this keyword…
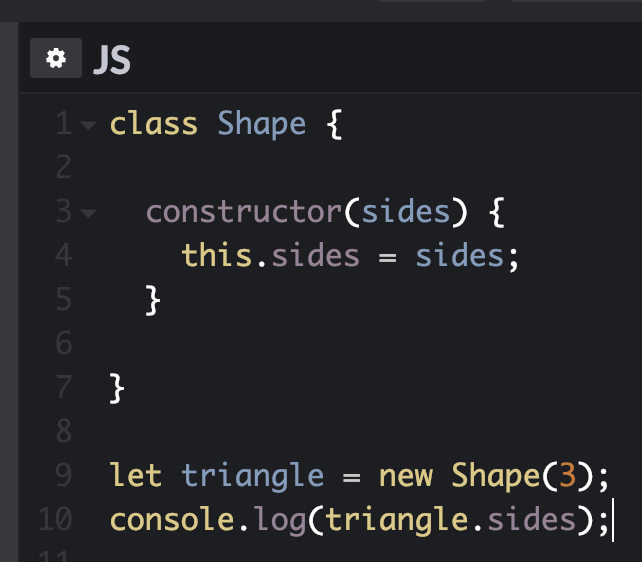
And you need to use the keyword new to create an instance of that class.
In Python, the equivalent is the __init__ magic method, which is technically an “initializer” instead of a “constructor”. Python’s real constructor is __new__.
class Shape():
def __init__(self, name):
self.name = name
It’s first parameter is always “self” followed by other initialization variables which it saves to class properties attached to “self” (instead of “this”). You don’t need to use the keyword new to create an instance.
Note that in Javascript class definition, no parenthesis after classname. In Python, yes parenthesis. By convention, name the classname in caps in both languages.
In Javascript, static methods and properties are preceded by the keyword “static” …
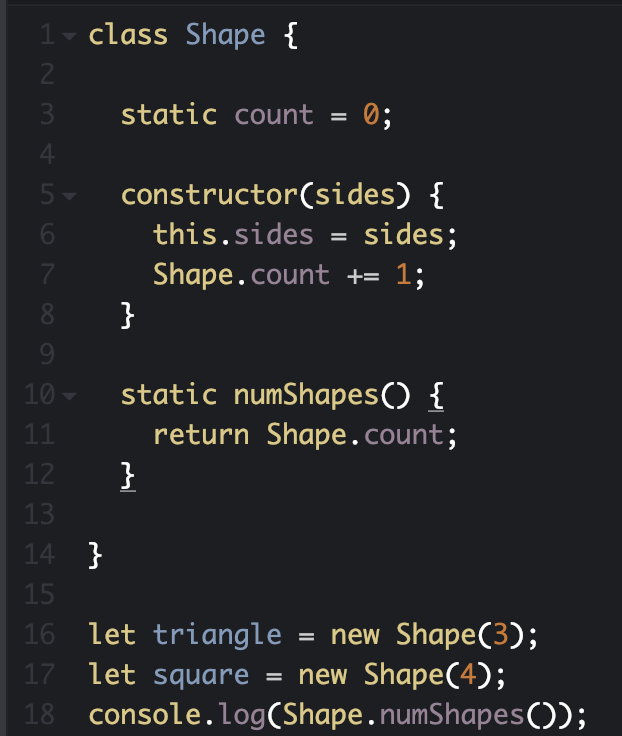
In Python, it is with the @staticmethod decorator …
class Shape():
def __init__(self, name):
self.name = name
@staticmethod
def say_something():
print("Hello from Shape")
It also has the @classmethod decorator which Javascript does not have.
In Javascript, use “get” and “set” to form getters and setters…
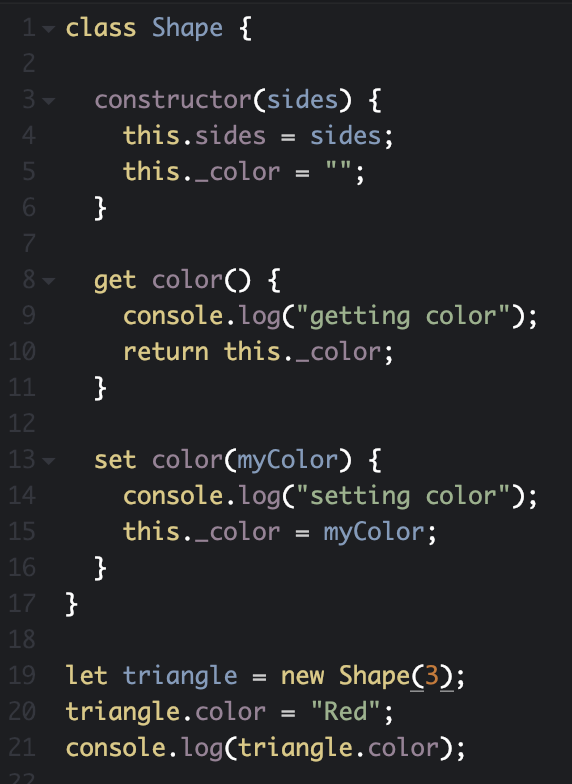
In Python, use @property and @your_property.setter instead.
In both, you can derive sub-classess. For Javascript, you use the keyword “extends” and you can call its parents constructor with “super”. For Python, you use parenthesis instead …
class Square(Shape):
def __init__(self, name, length):
super().__init__(name)
self.length = length
You also use “super” to access parent constructor, but in a slightly different way.
At current, there is no “private” in both Javascript and Python. Python has name-mangling. And Javascript “private” is in discussion.