Beginning Twig Templating Tutorial
Twig is a PHP server-side templating engine. Twig is being used in Drupal 8 and often used with Symfony 2. We’ll learn to run Twig in this beginning tutorial.
1. First download Twig from http://twig.sensiolabs.org/ While they want you to install Twig using Composer, you can download Twig from the link shown.

download twig
In this example, we are downloading Twig version 1.14.0.
2. You get a .tgz file (tarred-gzipped file), which you will have to uncompress and then untar. On Windows, you can use 7-Zip. Go into each of the extracted folder until you find the folder “Twig” that contains the Autoloader.php file. This is the Twig folder we care about. It is found inside the lib folder.
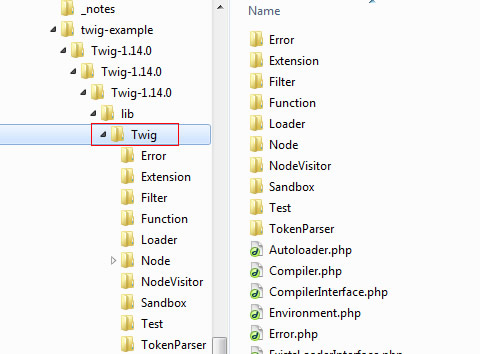
twig folder
3. Put “Twig” folder in the root of your PHP application folder. You need server running at least PHP 5.2.4 to run Twig.
4. In your PHP application folder, create an index.php file right next to your Twig folder.
5. Inside your index.php, include the Twig Autoloader.php file. This includes the Twig library into your PHP app. The autoloader will automatically load the other Twig files as needed so you don’t need to worry about including anything else.
<?php require_once 'Twig/Autoloader.php'; ?>
6. If you look inside Autoloader.php, you will see that it contains the class Twig_Autoloader with a static class function “register” which takes one optional parameter. So we call that to register our Twig autoloader.
<?php require_once 'Twig/Autoloader.php'; Twig_Autoloader::register(); ?>
7. Next we need to be able to load template files into Twig. Poking around in the Twig folder, we find a “Loader” folder which contains the file Filesystem.php. This is for the class Twig_Loader_Filesystem whose constructor takes “A path or an array of paths where to look for templates” (this was from the code comments). So let’s instantiate this Twig loader and pass it the path to our templates folder.
<?php require_once 'Twig/Autoloader.php'; Twig_Autoloader::register(); $loader = new Twig_Loader_Filesystem('templates'); ?>
8. We’ll create a folder called “templates” to hold our templates.
9. Next we pass this loader into our Twig environment which we create by doing …
<?php require_once 'Twig/Autoloader.php'; Twig_Autoloader::register(); $loader = new Twig_Loader_Filesystem('templates'); $twig = new Twig_Environment($loader); ?>
10. You see in Environment.php that the constructor of the class Twig_Environment takes an options array as its second argument. One of its option is to set up the cache. By default cache is disabled. To turn on cache in a production system, do this instead …
<?php
require_once 'Twig/Autoloader.php';
Twig_Autoloader::register();
$loader = new Twig_Loader_Filesystem('templates');
$twig = new Twig_Environment($loader, array('cache' => 'cache'
) );
?>
Note that on development environment, you might need to remove the cache in order to see changes whenever you change the template file.
11. That means we have to create a “cache” folder that is writable by the application (permission 755 will usually work). Your file system should now look like this …
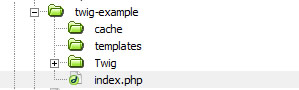
file system for twig
12. Now we are ready to start building our HTML page and using Twig. Add the basic html5 page below our PHP in index.php …
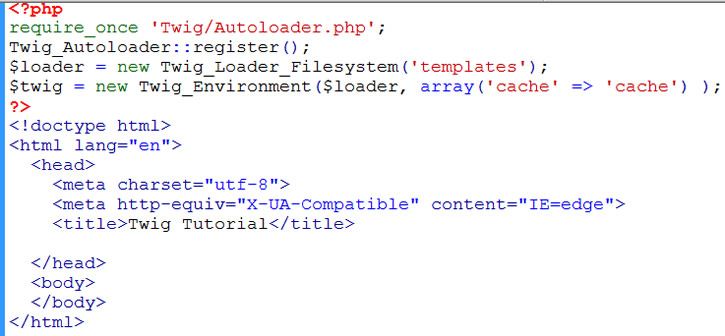
twig page
13. We are going to use a Twig template to display a “Hello” greeting. Within our “templates” folder, create a file called greeting.phtml (The phtml file extension indicates a template). But you can use the HTML extension such as greeting.html if you like.

twig template file
As you can see, this twig template file is just HTML but with a template variable interspersed. The template variable “name” is denoted by double-curly braces (just like in handlebarsJS).
14. We’ll have twig load this template …
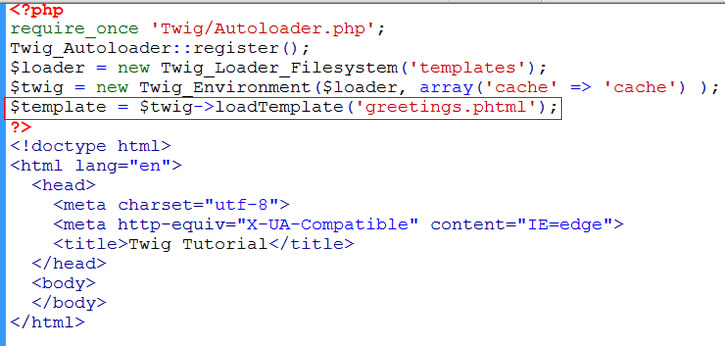
twig load template
15. This will return to us a template class which we can call its display function to display the template into our HTML. But first we have to feed our display function an array of parameters — one of which include our name variable…
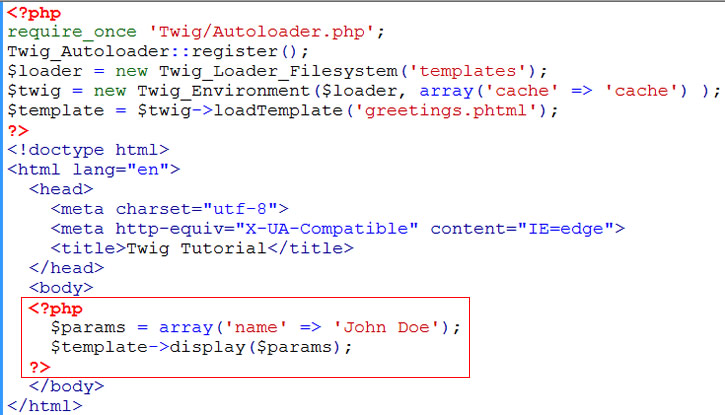
complete twig example
16. When rendered on our browser, you get …
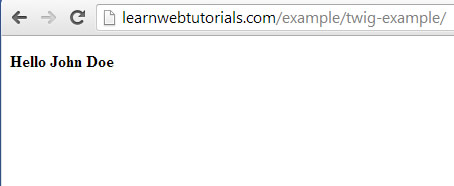
twig template output
Note that we did not need the echo statement here. $template->display() takes care of that.