Anatomy of a WordPress Plugin
To learn the anatomy of a WordPress plugin and how plugin works, we will start looking at a simply plugin, a plugin that comes pre-install with WordPress. It is the “Hello Dolly” plugin. In fact this plugin is there to show you an example of how to do a WordPress plugin.
First let’s see how it work. In WordPress Dashboard, go to “Plugins -> Installed Plugins” and make sure that the Hello Dolly plugin is activated. If not, click the “Activate” link. If you don’t see the “Activate” link and see the “Deactivate” link instead, that means that the plugin is activated.
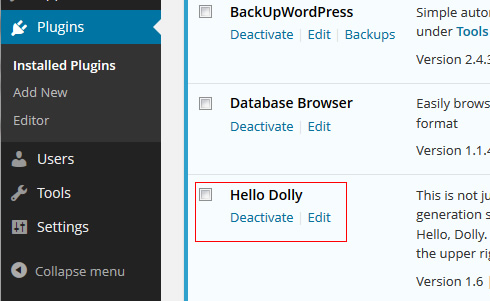
activate plugin
You should get the message “Plugin Activated” and you will start seeing a random Hello Dolly quote in the upper-right corner of your WordPress dashboard….
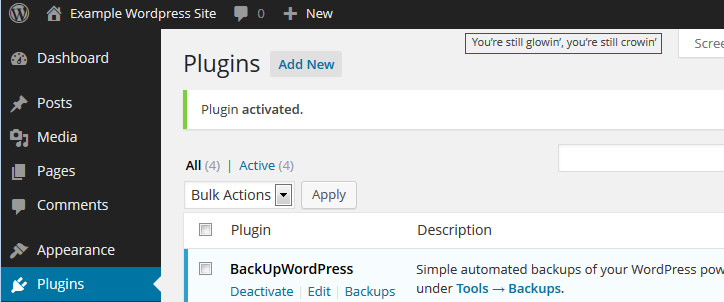
hello dolly quotes
Don’t worry, the Hello Dolly plugin only affects the WordPress admin backend. If you look at the front pages of the site that your user sees, there is no change.
Now let’s see how this WordPress Plugin Works
We open up the hood to look at the code by clicking on the “Edit” link in the Plugin Listing page (shown above).
You get an code editor showing the code for a file name “hello.php”. …
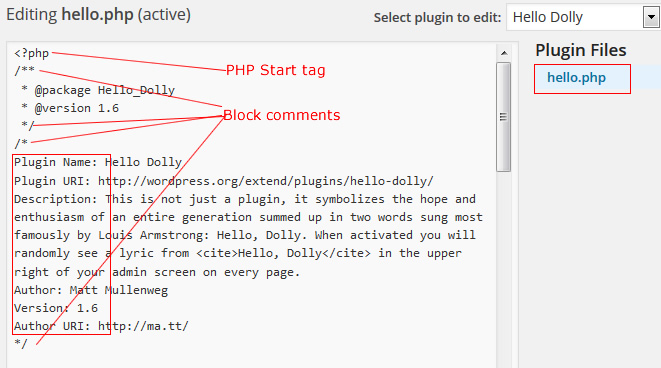
plugin code
This plugin is fairly simply so it consists of just this one PHP file. This hello.php file actually sits in /wp-content/plugins/hello.php if you look on your server. Although this plugin is not within its own folder, you will find that almost all other plugins will be within its own plugin sub-folder under the plugins directory.
The code start with the PHP start tag. The PHP ending tag is optional as long as the file ends in PHP code. In fact, it is preferable to leave it out. If you do have an ending PHP tag, make sure nothing after it.
Anyways, the top of the hello.php file start off with comments beggining with the header block. This is the PHPDoc file header block used to give an overview of what is contained in …
/** * @package Hello_Dolly * @version 1.6 */
WordPress documentation handbook states…
“Whenever possible, all WordPress files should contain a header block, regardless of the file’s contents – this includes files containing classes.”
Next comes the plugin documentation comments. What you put here is what will be shown in the plugin page …
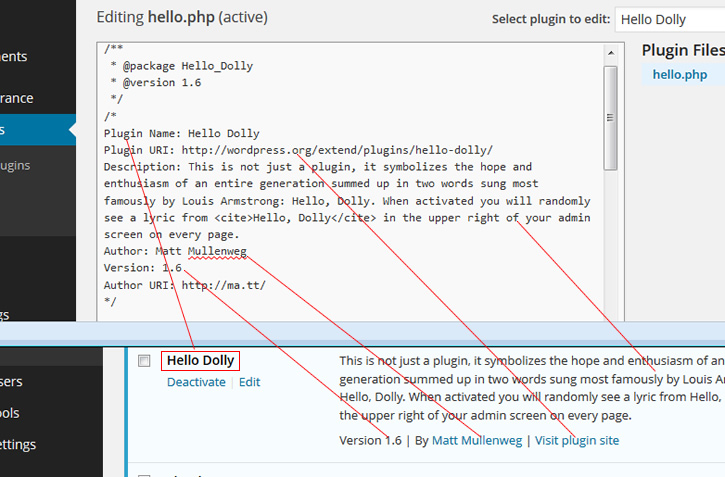
wordpress plugin documentation
See how that works?
“Plugin Name” declares the plugin name and is used by WordPress (among other things) to show it on the Plugins page.
Plugin URL is where you can find the plugin download link
Description is the description shown in the plugin page. You can give brief user instructions here.
Author tells who created the plugin, also shown in the Plugins page
Version tells plugin version, also shown in the Plugins page but also for determining updates
Author URI is the URI of the Author, generally used to supply an URL where the author can be contacted. It is the link destination of the author link shown in the plugin page.
Register WordPress Plugin Hooks
At the bottom of the file is where the action start with this code …
add_action( ‘admin_head’, ‘dolly_css’ );
The “add_action” call is known as an WordPress action hook. This line of code is known as registering hooks. Learn more about Action hooks. “admin_head” is the specific action hook that is being called. You can look it up on the WordPress Codex. It has to be spelled exactly like this. It is not an arbitrary name.
The codex writes that …
"admin_head is an action event and can be hooked by add_action hook.”
Whenever the <head> of an admin page loads (which is whenever the page loads), this hook is triggered and the “dolly_css” function is called. dolly_css is an arbitrarily name function which is defined higher up in the file…
// We need some CSS to position the paragraph function dolly_css() { // This makes sure that the positioning is also good for right-to-left languages $x = is_rtl() ? 'left' : 'right';
echo " <style type='text/css'> #dolly { float: $x; padding-$x: 15px; padding-top: 5px; margin: 0; font-size: 11px; } </style> "; }
This function adds some embedded CSS to the page. This declares the style for the lyrics of the Hello Dolly quote. The is_rtl() checks if the site is RTL (right to left language) or LTR (left to right language) and adapts the styles. The echo statement generates the dynamic styles used for the paragraph with the lyric.
But how does the Hello Dolly quote get displayed? It is through another action hook …
// Now we set that function up to execute when the admin_notices action is called add_action( 'admin_notices', 'hello_dolly' );
This hooks into the admin_notices event which according to the WordPress Codex is for…
“Notices displayed near the top of admin pages”
This hook registers the text generating function to be called on the admin panel. The hook triggers the function hello_dolly which is our function used to display the lyric.
The hello_dolly function is also defined in hello.php as the following …
// This just echoes the chosen line, we'll position it later function hello_dolly() { $chosen = hello_dolly_get_lyric(); echo "<p id='dolly'>$chosen</p>"; }
The hello_dolly display the lyrics by calling hello_dolly_get_lyrics() which return a random lyric. The returned lyric is displayed inside a paragraph with the echo statement.
The hello_dolly_get_lyrics() function has all the lines of the lyrics by stores all the lyrics in an string variable called $lyrics with each line of the lyrics separated by a “\n” newline character …
function hello_dolly_get_lyric() { /** These are the lyrics to Hello Dolly */ $lyrics = "Hello, Dolly Well, hello, Dolly It's so nice to have you back where you belong You're lookin' swell, Dolly I can tell, Dolly You're still glowin', you're still crowin' You're still goin' strong We feel the room swayin' While the band's playin' One of your old favourite songs from way back when So, take her wrap, fellas Find her an empty lap, fellas Dolly'll never go away again Hello, Dolly Well, hello, Dolly It's so nice to have you back where you belong You're lookin' swell, Dolly I can tell, Dolly You're still glowin', you're still crowin' You're still goin' strong We feel the room swayin' While the band's playin' One of your old favourite songs from way back when Golly, gee, fellas Find her a vacant knee, fellas Dolly'll never go away Dolly'll never go away Dolly'll never go away again";
// Here we split it into lines $lyrics = explode( "\n", $lyrics );
// And then randomly choose a line return wptexturize( $lyrics[ mt_rand( 0, count( $lyrics ) - 1 ) ] ); }
By splitting at the newline “\n”, the explode function converts the $lyrics variable into an array, with each element of the array containing one line of the lyrics.
mt_rand is used to return a random line from the $lyrics array. And wptexturize transforms quotes to smart quotes, apostrophes, dashes, ellipses, the trademark symbol, etc.
The plugin is a good starting place to learn. Plugins can definitely get much more complex.